In the latest project at Nimbus Academy, trainees explored the dynamic realm of Streamlit—a versatile Python module that transforms scripts into interactive web applications. This article delves into the nuances of managing reactivity in Streamlit, offering insights into persisting variables and designing with statefulness in mind.
Understanding Streamlit Reactivity
Streamlit’s automatic script re-execution feature can be both a boon and a challenge for developers. While it is core the Streamlit development flow (“type some code, save it, try it out live, then type some more code, save it, try it out, and so on”) managing what updates when can be tricky.
Streamlit reruns a script from top to bottom every time a user interacts with a widget in the app. This means that building a page refresh button is as simple as making a button called “refresh” that otherwise does nothing:
import streamlit as st
import random
st.button("refresh") # functioning refresh button
my_list = ["a", "b", "c"]
st.write(random.choice(my_list)) # makes a new random choice
Persisting Variables with Streamlit Session State
Question: What happens to the value of ‘x’ in this script after clicking the refresh button?
import streamlit as st
st.header("Incrementing X")
x = 5 # define x
st.write("The value of x is", x)
x +=1 # increment x by 1
st.write("The new value of x is", x)
st.button("refresh")
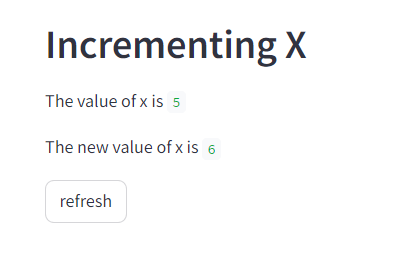
Answer: On every refresh, ‘x’ resets to 5.
The key to preserving variable values lies in understanding Streamlit’s session state. When a browser tab connects to a Streamlit app, a new session is created. By utilizing the session_state
attribute, developers can persist data between page refreshes.
For instance, consider a scenario where a variable ‘x’ needs to be incremented every time the page refreshes.
import streamlit as st
# x is defined once the session is started
if 'x' not in st.session_state:
st.session_state.x = 5
st.header("Incrementing X")
st.write("The value of x is", st.session_state.x)
st.session_state.x +=1 # increment x by 1
st.button("refresh")
Designing Streamlit Applications with Statefulness in Mind
Optimizing Streamlit applications for both reactivity and statefulness is essential for user satisfaction. Here are some best practices to consider:
- Identify and Persist: From the start, pinpoint variables requiring persistence, initializing them in the
session_state
. This ensures they endure user interactions without resetting. - Utilize Reactive Widgets: Leverage Streamlit’s interactive widgets in conjunction with session state to create dynamic, user-responsive applications. Widgets like sliders and buttons can trigger stateful updates.
- Feedback and Loading Indicators: Employ session state to toggle loading indicators, providing users with feedback during time-consuming operations. This enhances user experience and manages expectations.